"Implement an application that will enable a user to specify a color and will propagate to all sensors a command to blink in the user-specified color. The user will issue the command from a host computer attached to a base station node. The base station will broadcast the command, and the sensors will use the mesh networking protocol provided by the API to propagate the command to the entire network. "[1]
Analysis:
We can divide this task into two individual tasks: 1. The base station broadcasts the "Change Color" command; 2. free-range SPOT that can directly communicate with the base station sends the same command to the other SPOTs that are beyond the base station's radio range.The core part of this task is to use communication APIs and debug all applications. We need import com.sun.spot.io.j2me.radiogram to realize communication between base station and free-range SPOTs or among free-range SPOTs.
There are two ways to propagate the command in the entire network.
1. A free-range SPOT continuously resend the same command after it receive the "Change Color" command. In this case we need a good algorithm to avoid command conflicts because of communication time delay.
Free-Range SPOT Side Work Flow

After a SPOT receives a "Change Color" command, it will parse this command to get the source's "NodeLevel" nlA. If this value is less than its own "NodeLevel" nlB, we think the source node could be its ParentNode so that we blink LEDs in this given color and set "NodeLevel" nlB as "NodeLevel" nlA + 1. Otherwise, we need to check whether its previous ParentNode is still alive. If its ParentNode is dead, we set the source node as its new ParentNode by changing "NodeLevel" nlB to "NodeLevel" nlA + 1.
There is a Hashtable inside to maintain all SPOT nodes' "Alive" information that current node can reach. When the SPOT get any "Alive" message from the other SPOTS, it just modifies the relevant value.
2. Turn a free-range SPOT as a mesh router.
When the base station broadcasts the command, this command will go everywhere in the whole network. In order to realize this, we need to execute the following command to set SPOT.
"ant set-system-property –Dkey=spot.mesh.enable –Dvalue=true"
In addition, we need to set the number of hops when we create the connection like this:
((RadiogramConnection)dgConnection).setMaxBroadcastHops(4);
Note: Users use command line to specify different color. Currently, we support two ways to input:LEDColor and RGB so that users can just provide colors' name or rgb value.
Implementation:
In SPOT host side, I used two threads to execute sending and receiving message. The following code can demonstrates the communication process:
// Create a DatagramConnection
DatagramConnection dgConnection = null;
Datagram dg = null;
// Datagram Connection Configuration
dgConnection = (DatagramConnection) Connector.open("radiogram://broadcast:37");
//Set the number of hops
((RadiogramConnection)dgConnection).setMaxBroadcastHops(4);
dg = dgConnection.newDatagram(dgConnection.getMaximumLength());
// Send Messages
dg.reset();
dg.writeUTF(strMessage);
dgConnection.send(dg);
// Receive Messages
dg.reset();
dgConnection.receive(dg);
strMessage = dg.readUTF();
I used "regular expression" to parse the commands that users input. The standard command format is like below:
1. name ColorName E.g. name red name blue
Regular Expression:
name (blue|chartreuse|cyan|green|magenta|mauve|orange|puce|
red|turquoise|white|yellow){1}$
2. rgb value value value E.g. rgb 100 100 100 rgb 255 0 0
Regular Expression:
rgb( ([0-9]|[1-9][0-9]|1[0-9][0-9]|2[0-4][0-9]|25[0-5])){3}$
In free-range SPOT side, I reused color-control code finished in Task 2 to change LEDs' color and propagate the commands to the other SPOTs by "broadcast" mode.
Testing:
I designed ten test cases to simulate different network structure. But we can satisfy this task's requirement if these applications can pass through some typical scenarios. So I chose a couple of test cases.
Different Test Scenarios(4 Free-Range SPOTs)

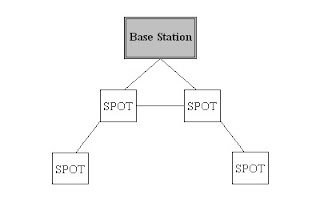




Difficulty and Challenge:
In this task, testing brought me a lot trouble because it's difficult to check the intermediate value hidden in a free-range SPOT. So I spent a lot of time on testing. This task is much harder than the previous one. I drew a work flow chart to help myself deeply figure out the communication process among SPOTs.
Problem: Can't use the Console to run a host application
1. To attach require jars' path to the current classpath
2. To enable the base station by executing the command "ant selectbasestation"
3. To run "ant host-run"
Time Distribution:
Task | Description | Deadline | End Date | Total Time (hrs) |
3 | Transferring a COLOR command in the entire SPOT network | 06/20/2008 | 06/20/2008 | 32.9 |
Sub Task | Time | |||
Requirement Analysis | 1.5 | |||
Study 2 demos related this task again | 4 | |||
Create a SPOT Host Application and implement “broadcast” functionality | 2 | |||
Finish the work flow on free-range SPOT side | 4.5 | |||
Create a SPOT application and implement all functionalities | 3.2 | |||
Simple Test | 8 | |||
Create test scenarios | 1.2 | |||
System Test | 4 | |||
Turn a SPOT as a mesh router and test | 1 | |||
Miscellaneous Task (updating the blog, documents and so on) | 3.5 |
[1]Quoted from the section "Task 3" of Class Requirement Document
Written by Professor Sami Rollins